Preparing for PHP interview: Everything you wanted to know about interfaces, compatibility signature and not afraid to learn
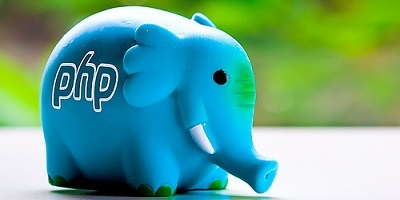
It would seem — what could be simpler interface? "Like a class but not a class that cannot be instantiated, but rather a contract for future classes, it contains the headers of the public methods of" — aren't these words you often meet in the interview on the attendant question of what interface?
However, it is not so simple as it may seem to a novice programmer in PHP. The usual analogy does not work, the guide for language fool you, the code lurk unexpected pitfalls...
The previous three parts:
the
-
the
- Preparing for interview for PHP: the keyword "static" the
- Preparing for interview for PHP: a pseudo-type "callable" the
- Preparing for interview for PHP: All about iteration, and a little about the pseudo-type "iterable"
the
That may contain interface?
It is obvious that public methods with no implementation: immediately after the header (signature) method must end with a semicolon:
the
interface SomeInterface
{
public function foo();
public static function bar(Baz $baz);
}
Slightly less obvious (but is mentioned in the manual) the fact that an interface can contain constants (of course, only public!):
the
interface SomeInterface
{
public const STATUSES = [
'OK' => 0,
'ERROR' => 1,
];
}
if (SomeInterface::STATUSES['OK'] === $status) {
// ...
}
Why constants in interfaces are not widespread in the industrial code, although sometimes used? The reason is that they cannot be overridden in an interface inheriting from or in a class implementing this interface. Interface constants — the const constants in the world :)
the
What can not contain the interface?
Nothing more can. In addition to the headers of public methods and public constants.
It is impossible to include in the interface:
the
-
the
- Any properties the
- non-public methods the
- Methods with the implementation the
- non-public constants
In fact, strictly speaking, it and interface!
the
Compatibility of method signatures
For further study of the interfaces we need to know about the most important concept that is unjustly neglected in the PHP manual: about the concept of "compatibility of signatures."
A signature is a description of the function (method) that includes:
the
-
the
- access Modifier the
- the name of the function (method) the
- List of arguments where each argument is specified:
the-
the
- Type
the Name
the - default Value the
- or operator "three points"
the
Examples:
the
function ();
public function foo($arg = null);
protected function sum(int $x, int $y, ...$args): int;
Suppose we have two functions, A and B.
The signature of the function B is considered to be compatible with A (the order is important, the relation is asymmetrical!) in the strict sense if:
They are identical
Trivial case, there is nothing to comment on.
B adds A default arguments
A:
the
function foo($x);
compatible B:
the
function foo($x, $y = null);
function foo($x, ...$args);
B narrows the range of values A
A:
the
function foo(int $x);
compatible B:
the
// A is allowed a refund of any values in B, this area is narrowed only to integers
function foo(int $x): int;
Now, when we introduced these three simple compatibility rules definitions will be much easier to understand the further details associated with interfaces.
the
Inheritance interfaces
Interfaces can inherit from each other:
the
interface First
{
public const PI = 3.14159;
public function foo(int $x);
}
interface Second
extends First
{
public const E = 2.71828;
}
assert(3.14159 === First::PI);
assert(true === method_exists(First::class, 'foo'));
assert(3.14159 === Second::PI);
assert(2.71828 === Second::E);
assert(true === method_exists(Second::class, 'foo'));
assert(true === method_exists(Second::class, 'bar'));
Interface-the heir receives from an interface ancestor inherited all defined in the ancestor methods, and constants.
In the interface of the inheriting, you can override a method from a parent interface. But only under the condition that either his signature will exactly match the signature of the parent or will be compatible (see previous section):
the
interface First
{
public function foo(int $x);
}
interface Second
extends First
{
// So it is possible, but pointless
public function foo(int $x);
// Shouldn't, fatal error Declaration of must be compatible
public function foo(int $x, int $y);
// So it is possible, because this signature is compatible with the parent - we just added an optional argument
public function foo(int $x, int $y = 0);
// That's right, all arguments after "..." are optional
public function foo(int $x, ...$args);
// And so can also be
public function foo(int $x, ...$args): int;
}
the
If PHP multiple inheritance?
If you are asking this question, feel free to reply: Yes. An interface can inherit from several other interfaces.
Now you've seen it all:
the
interface First
{
public function foo(int $x);
}
interface Second
{
public function bar(string $s);
}
interface Third
extends First, Second
{
public function baz(array $a);
}
assert(true === method_exists(Third::class, 'foo'));
assert(true === method_exists(Third::class, 'bar'));
assert(true === method_exists(Third::class, 'baz'));
Rules of conflict resolution signatures of the methods when multiple inheritance is exactly the same as we have seen above:
— or signatures match exactly.
any method signature of the interface mentioned in the list of ancestors of the first, must be compatible with the signature of the second ancestor (Yes, the order of mention is important, but it is a very rare case, just don't ever take it into consideration)
the
implementation details interfaces
Actually, after what you have seen, this is not the intricacies and little nuances.
First, indeed, the class inheritance from an interface is called realization. The point is that you don't just inherit the methods and constants, but have to implement those methods that match the signatures, fill them with this code:
the
interface IntSumInterface
{
public function sum(int $x, int $y): int;
}
interface IntMultInterface
{
public function mult(int $x, int $y): int;
}
class Math
implements IntSumInterface, IntMultInterface
{
public function sum(int $x, int $y): int
{
return $x + $y;
}
public function mult(int $x, int $y): int
{
return $x * $y;
}
}
An important aspect that distinguishes the interface implementation from inheritance from another class is able to implement in one class multiple interfaces at once.
What if in the different interfaces implemented by the class, will the same method (with same name)? See above — as well as when you inherit interfaces from each other must respect the principle of compatibility of the signature.
And Yes. Don't believe the manual, which says:
the signatures of the methods in a class implementing the interface must exactly match the signature used in the interface, otherwise it will caused a fatal error.
The class implementing the interface must use the exact same method signatures as are defined in the interface. Not doing so will result in a fatal error.
It is not so, applies the same rule compatibility:
the
interface SomeInterface
{
public function sum(int $x, int $y);
}
class SomeClass
implements SomeInterface
{
public function sum(int $x, int $y): int
or
public function sum(int $x, int $y, int $z = 0): int
or even
public function sum(int $x, int $y, ...$args): int
{
// method implementation
}
}
the
Interface is a class? Pro et Contra
Actually, no. The interface is defined, it is different from a class at least the fact that you cannot create "the instance interface".
And actually, Yes, in PHP they have a lot in common:
-
the
- Interfaces, like classes, can be in a namespace. the
- Interfaces, like classes, can be loaded through the startup mechanism. Function startup will be given a full interface name (with the namespace). the
- Interface of a class can participate right instanceof the
- Interface of a class can be specified as the type in the type-hinting (specifying the type of the argument or return value of a function)
the
something to read on the night before an important job interview?
Of course, the manual for the language:
the
-
the
- php.net/manual/ru/language.oop5.interfaces.php the
- php.net/manual/ru/language.oop5.constants.php the
- php.net/manual/ru/language.constants.predefined.php
But it is much better to read heavy technical texts last night and in advance.
A systematic approach to self-education in programming is very important. And, in my opinion, a good idea in the beginning, IT helps to structure self-study webinars and short courses. That is why I recommend (and a few modestly advertised), even experienced developers to attend one-time webinars and training courses — the result with the right combination of courses and self study is always there!
Success at interview and in work!
Комментарии
Отправить комментарий